C言語において文字列を比較する方法はさまざまあります。
文字列を部分一致で比較する方法と文字列を完全一致で比較する方法について解説します。
これにより異なる状況に応じて適切な比較手法を選択できるようになります。
文字列の比較を部分一致で判定する
部分一致の比較には、独自の関数を作成する必要があります。
以下は、部分一致を判定するための関数の例です。
#include <stdio.h>
#include <string.h>
// 部分一致を判定する関数
int isPartialMatch(const char *str1, const char *str2) {
while (*str1 != '#include <stdio.h>
#include <string.h>
// 部分一致を判定する関数
int isPartialMatch(const char *str1, const char *str2) {
while (*str1 != '\0' && *str2 != '\0') {
if (*str1 == *str2) {
++str1;
}
++str2;
}
return (*str1 == '\0');
}
int main() {
// 部分一致を判定
if (isPartialMatch("hello", "he")) {
printf("文字列は部分一致しています。\n");
} else {
printf("文字列は部分一致していません。\n");
}
return 0;
}
' && *str2 != '#include <stdio.h>
#include <string.h>
// 部分一致を判定する関数
int isPartialMatch(const char *str1, const char *str2) {
while (*str1 != '\0' && *str2 != '\0') {
if (*str1 == *str2) {
++str1;
}
++str2;
}
return (*str1 == '\0');
}
int main() {
// 部分一致を判定
if (isPartialMatch("hello", "he")) {
printf("文字列は部分一致しています。\n");
} else {
printf("文字列は部分一致していません。\n");
}
return 0;
}
') {
if (*str1 == *str2) {
++str1;
}
++str2;
}
return (*str1 == '#include <stdio.h>
#include <string.h>
// 部分一致を判定する関数
int isPartialMatch(const char *str1, const char *str2) {
while (*str1 != '\0' && *str2 != '\0') {
if (*str1 == *str2) {
++str1;
}
++str2;
}
return (*str1 == '\0');
}
int main() {
// 部分一致を判定
if (isPartialMatch("hello", "he")) {
printf("文字列は部分一致しています。\n");
} else {
printf("文字列は部分一致していません。\n");
}
return 0;
}
');
}
int main() {
// 部分一致を判定
if (isPartialMatch("hello", "he")) {
printf("文字列は部分一致しています。\n");
} else {
printf("文字列は部分一致していません。\n");
}
return 0;
}
この例では、一方の文字列の各文字が他方の文字列内で順番に見つかるかどうかを確認しています。
もし一方の文字列の全ての文字が見つかれば、部分一致と判定されます。
広告
文字列の比較を完全一致でチェックする
完全一致の比較には、strcmp関数を使用します。以下はその基本的な例です。
#include <stdio.h>
#include <string.h>
int main() {
// 文字列を比較
if (strcmp("hello", "hello") == 0) {
printf("文字列は完全に一致しています。\n");
} else {
printf("文字列は一致していません。\n");
}
return 0;
}
strcmpは、比較対象の文字列が等しい場合には0を返します。
この0を条件として使って、文字列が完全に一致しているかどうかを判定します。
以下記事でstrcmp関数を利用しないで比較をする方法についても紹介しています。
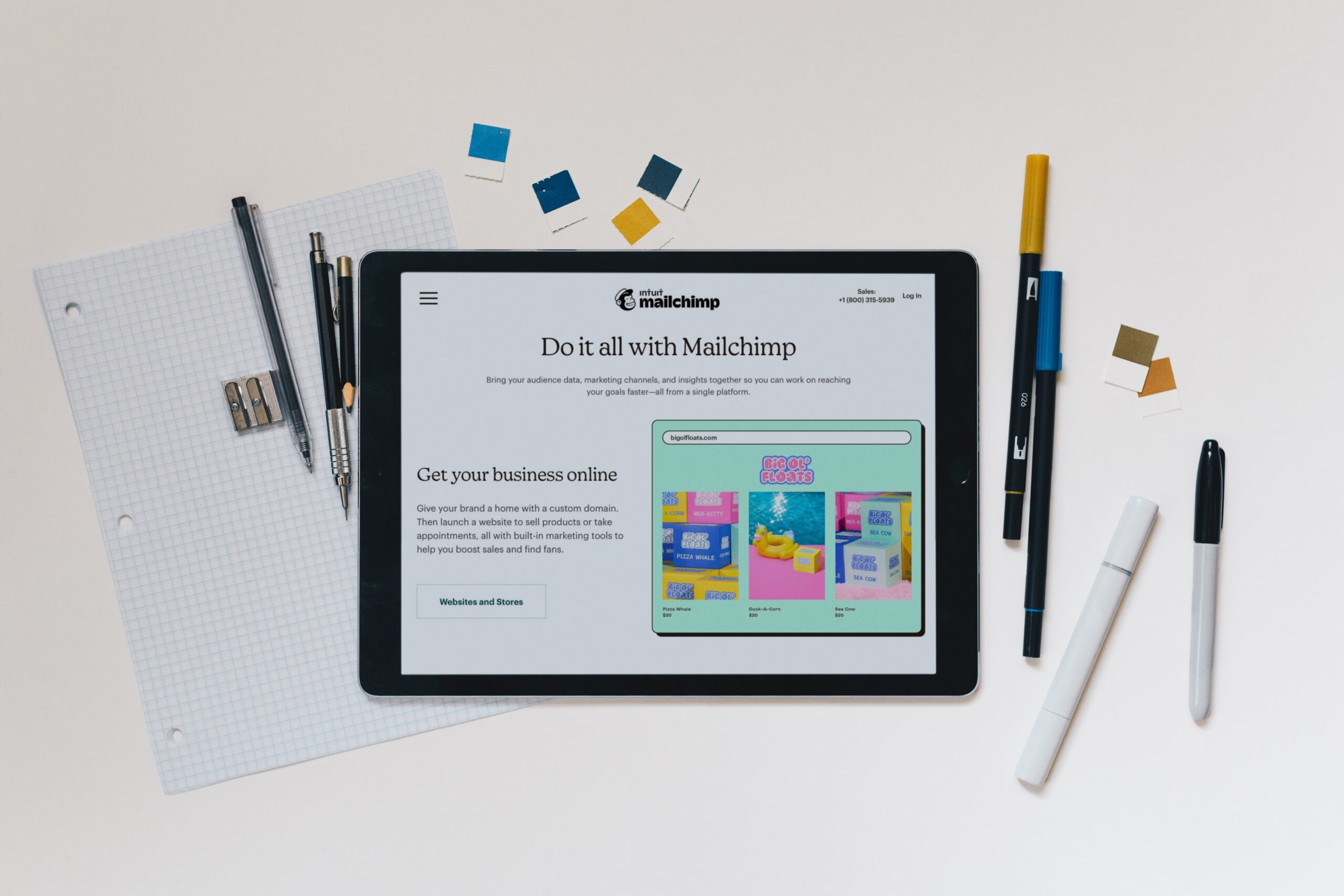
[C言語]strcmpを使わないで文字列を比較する!基本的なチェック方法
C言語において、文字列を比較する方法にはいくつかのアプローチがあります。ここでは、strcmpを使わないで文字列を比較する方法と、strcmpを使った比較方法について解説します。これにより、異なるアプローチを理解し、柔軟に文字列比較を行うスキルを身につけましょう。
広告
まとめ
C言語において文字列の比較には、完全一致の場合にはstrcmpを使用し、
部分一致の場合には独自の関数を作成することが一般的です。
どちらも使い方を理解しておくと、異なる比較ニーズに対応できるようになります。